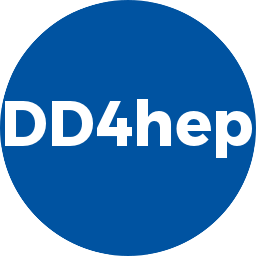 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
13 #ifndef DDREC_DETECTORDATA_H
14 #define DDREC_DETECTORDATA_H
20 #include <boost/variant.hpp>
489 std::map<dd4hep::CellID , std::vector<dd4hep::CellID > >
sameLayer ;
492 std::map<dd4hep::CellID , std::vector<dd4hep::CellID > >
prevLayer ;
495 std::map<dd4hep::CellID , std::vector<dd4hep::CellID > >
nextLayer ;
516 template <
typename T>
517 const T&
get(
const std::string&
key)
const {
520 throw std::runtime_error{
"Key "+
key+
" not found"};
522 return boost::get<T>(it->second);
525 template <
typename T>
529 throw std::runtime_error{
"Key "+
key+
" not found"};
531 return boost::get<T>(it->second);
534 template <
typename T>
540 return boost::get<T>(it->second);
543 template <
typename T>
544 void set(
const std::string&
key, T value) {
559 #endif // DDREC_DETECTORDATA_H
double distanceSensitive
The distance of the petal sensitive from the z-axis.
std::map< std::string, boost::variant< double, int, std::string, bool > > variantParameters
double cellSize0
cell size along the first axis where first is either along the beam (BarrelLayout) or up (EndcapLayou...
double sensitive_thickness
Thickness of the sensitive element (e.g. scintillator)
double thicknessSensitive
The thickness of the petal sensitive.
double pitchStrip
strip pitch (if applicable )
double outer_thickness
Distance between the center of the sensitive element and the outermost face of the layer.
double angleStrip
strip stereo angle (if applicable )
std::vector< LayerLayout > layers
double rInner
inner r at start of section
StructExtension(const StructExtension< T > &t)
double distanceSupport
The distance of the petal support from the z-axis.
std::vector< Layer > layers
double zHalfSupport
The half length of the ladder support in z.
double widthInnerSensitive
The inner width of the petal sensitive.
double distanceSensitive
The distance of the ladder sensitive from the origin (IP).
double distanceSupport
The distance of the ladder support from the origin (IP).
double inner_nInteractionLengths
Absorber material in front of sensitive element in the layer, units of radiation lengths.
double outer_nRadiationLengths
Absorber material in behind of sensitive element in the layer, units of radiation lengths.
double angleStrip
strip stereo angle (if applicable )
double widthInnerSupport
The inner width of the petal support.
double phi0
phi0 of layer: potential rotation around normal to absorber plane, e.g. if layers are 'staggered' in ...
double driftLength
driftLength in z (half length of active volume)
double rMaxReadout
outer r of active volume
double widthSupport
The width of the ladder support.
double rInnerShell
The inner radius of the support shell.
LayoutType layoutType
type of layout: BarrelLayout or EndcapLayout
double inner_nRadiationLengths
Absorber material in front of sensitive element in the layer, units of radiation lengths.
double gap0
Gap between modules(eg. stave gap) in the phi-direction.
std::map< dd4hep::CellID, std::vector< dd4hep::CellID > > prevLayer
map of all neighbours in the previous layer
double lengthSensor
sensor length (if divided in sensors)
int ladderNumber
The number of ladders in the layer.
double gap2
Gap between modules(reserved for future use) e.g in the r-direction.
enum for encoding the sensor type in typeFlags
StructExtension< NeighbourSurfacesStruct > NeighbourSurfacesData
std::map< std::string, double > doubleParameters
double offsetSupport
The offset of the ladder support, i.e. the shift in the direction of increasing phi,...
void set(const std::string &key, T value)
double zHalfSensitive
The half length of the ladder sensitive in z.
bool isSymmetricInZ
if true the sections are repeated at negative z
double petalHalfAngle
half angle covered by petal
StructExtension< LayeredCalorimeterStruct > LayeredCalorimeterData
double thicknessSensitive
The thickness of the ladder sensitive from the origin (IP).
double thicknessSupport
The thickness of the petal support.
double lengthSensitive
The radial length of the petal sensitive.
double cellSize1
second cell size, perpendicular to the first direction cellSize0 and the depth of the layers.
Handle class describing a detector element.
double lengthSupport
The radial length of the petal support.
double gapShell
The length of the gap in mm (gap position at z=0).
double zPosition
z-position of layer ( z-position of middle axis )
double innerWallThickness
thickness of the inner wall (field cage)
double gap1
Gap between modules(eg. middle stave gap) in the z-direction.
double rOuter
outer r at start of section
double zHalf
half length of the TPC
StructExtension(const T &t)
std::vector< Section > sections
std::vector< LayerLayout > layers
double zHalfShell
The half length (z) of the support shell (w/o gap) - 0. if no shell exists.
double lengthStrip
length of the strips (if applicable )
double widthStrip
width of the strips (if applicable )
double offsetSensitive
The offset of the ladder sensitive, i.e. the shift in the direction of increasing phi,...
double widthOuterSensitive
The outer width of the petal sensitive.
bool contains(const std::string &key) const
StructExtension< ConicalSupportStruct > ConicalSupportData
double widthStrip
width of the strips (if applicable )
double distance
distance from Origin (or the z-axis) to the inner-most face of the layer
double absorberThickness
thickness of the absorber part of the layer. Consider using inner/outer_nRadiationLengths and inner/o...
double widthSensitive
The width of the ladder sensitive.
int petalNumber
The number of petals in the layer.
const T & get(const std::string &key) const
double thicknessSupport
The thickness of the ladder support from the origin (IP).
double pitchStrip
strip pitch (if applicable )
StructExtension< ZDiskPetalsStruct > ZDiskPetalsData
StructExtension< ZPlanarStruct > ZPlanarData
double outerWallThickness
thickness of the outer wall (field cage)
std::bitset< 32 > typeFlags
Bit flag describing sensor type - use enum SensorType to access the bits.
double rMinReadout
inner r of active volume
StructExtension(const DetElement &d)
int sensorsPerPetal
number of sensor per petal
double padWidth
fixed pad width ( at middle of row)
std::map< dd4hep::CellID, std::vector< dd4hep::CellID > > sameLayer
map of all neighbours in the same layer
int sensorsPerLadder
number of sensor per ladder
double phi0
azimuthal angle of vector defined by the Z-axis to first petal x-positive, y-positive edge
Namespace for the AIDA detector description toolkit.
double rMin
inner radius of the TPC
std::ostream & operator<<(std::ostream &io, const DCH_info &d)
double inner_thickness
Distance between the innermost face of the layer (closest to IP) and the center of the sensitive elem...
LayoutType
enum for encoding the sensor type in typeFlags
StructExtension< FixedPadSizeTPCStruct > FixedPadSizeTPCData
double zPos
z position at start of section
double maxRow
maximum number of rows
double rOuterShell
The outer radius of the support shell.
double widthOuterSupport
The outer width of the petal support.
double outer_nInteractionLengths
Absorber material in behind of sensitive element in the layer, units of radiation lengths.
double lengthStrip
length of the strips (if applicable )
T & get(const std::string &key)
double rMax
outer radius of the TPC
std::map< dd4hep::CellID, std::vector< dd4hep::CellID > > nextLayer
map of all neighbours in the next layer
double phi0
Azimuthal angle of the (outward pointing) normal of the first ladder.
double padHeight
pad height of readout rows
double padGap
gap between pads
T value_or(const std::string &key, T alternative) const
double zMinReadout
start z of active Volume (typically cathode half thickness)
StructExtension(const StructExtension< T > &t, const DetElement &)