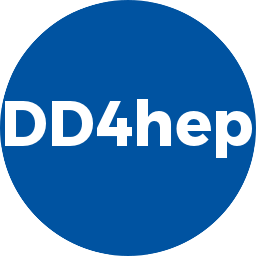 |
DD4hep
1.31.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
13 #ifndef DDREC_DDGEAR_H
14 #define DDREC_DDGEAR_H
19 #include "gear/GEAR.h"
20 #include "gearimpl/GearParametersImpl.h"
21 #include "gearimpl/SimpleMaterialImpl.h"
22 #include "gear/GearMgr.h"
25 class GearParametersImpl ;
43 gear::GearParametersImpl*
_gObj ;
53 GearHandle( gear::GearParametersImpl* gearObj,
const std::string& nam ) :
_gObj( gearObj ) ,
_name( nam ) {}
69 gear::GearParametersImpl* obj =
_gObj ;
76 void addMaterial(
const std::string nam,
double A,
double Z,
double density,
double radLen,
double intLen){
78 _materials.push_back( gear::SimpleMaterialImpl (nam, A, Z, density, radLen, intLen) ) ;
gear::GearParametersImpl * gearObject()
GearHandle(const DetElement &)
gear::GearParametersImpl * takeGearObject()
GearHandle(const GearHandle &, const DetElement &)
gear::GearParametersImpl * _gObj
const std::string & name()
std::vector< gear::SimpleMaterialImpl > _materials
Handle class describing a detector element.
gear::GearMgr * createGearMgr(Detector &description, const std::string &pluginName="GearForILD")
const std::vector< gear::SimpleMaterialImpl > & materials()
get all materials assigned to this wrapper
void addMaterial(const std::string nam, double A, double Z, double density, double radLen, double intLen)
add a SimpleMaterial object
Namespace for the AIDA detector description toolkit.
The main interface to the dd4hep detector description package.
GearHandle(gear::GearParametersImpl *gearObj, const std::string &nam)