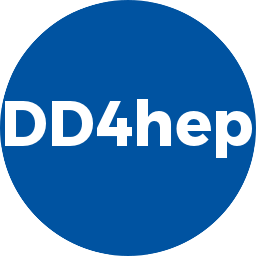 |
DD4hep
1.32.1
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
22 std::string _visLevel(
int lvl) {
24 std::snprintf(text,
sizeof(text),
"%d",lvl);
49 return dd4hep::setPrintLevel(level);
54 int old_value = visLevel;
61 if ( !m_condMgr.isValid() ) {
62 const void* argv[] = {
"-handle",&m_condMgr,0};
63 if ( 1 != apply(
"DD4hep_ConditionsManagerInstaller",2,(
char**)argv) ) {
64 except(
"DD4hepUI",
"Failed to install the conditions manager object.");
66 if ( !m_condMgr.isValid() ) {
67 except(
"DD4hepUI",
"Failed to access the conditions manager object.");
77 m_detDesc.fromXML(fname, m_detDesc.buildType());
85 if ( !m_alignMgr.isValid() ) {
86 const void* argv[] = {
"-handle",&m_alignMgr,0};
87 if ( 1 != apply(
"DD4hep_AlignmentsManagerInstaller",2,(
char**)argv) ) {
88 except(
"DD4hepUI",
"Failed to install the alignment manager object.");
90 if ( !m_alignMgr.isValid() ) {
91 except(
"DD4hepUI",
"Failed to access the alignment manager object.");
99 return m_detDesc.apply(factory, argc, argv);
104 return m_detDesc.fromXML(fname, type);
109 drawSubtree(
"/world");
114 redrawSubtree(
"/world");
119 std::string vis = _visLevel(visLevel);
120 const void* av[] = {
"-detector", path,
"-option",
"ogl",
"-level", vis.c_str(), 0};
121 m_detDesc.apply(
"DD4hep_GeometryDisplay", 2, (
char**)av);
126 std::string vis = _visLevel(visLevel);
127 const void* av[] = {
"-detector", path,
"-option",
"oglsame",
"-level", vis.c_str(), 0};
128 m_detDesc.apply(
"DD4hep_GeometryDisplay", 4, (
char**)av);
134 const void* av[] = {
"-positions",
"-pointers",0};
135 return m_detDesc.apply(
"DD4hep_VolumeDump",2,(
char**)av);
137 return m_detDesc.apply(
"DD4hep_VolumeDump",argc,argv);
142 const void* args[] = {
"--detector", path ? path :
"/world", 0};
143 return m_detDesc.apply(
"DD4hep_DetectorVolumeDump",2,(
char**)args);
148 const void* args[] = {
"--detector", path ? path :
"/world",
"--materials",
"--shapes", 0};
149 return m_detDesc.apply(
"DD4hep_DetectorVolumeDump",4,(
char**)args);
154 const void* args[] = {
"--detector", path ? path :
"/world", 0};
155 return m_detDesc.apply(
"DD4hep_DetectorDump",2,(
char**)args);
161 const void* av[] = {
"-output",file_name,0};
162 return m_detDesc.apply(
"DD4hep_Geometry2ROOT",2,(
char**)av);
164 printout(WARNING,
"DD4hepUI",
"++ saveROOT: No valid output file name supplied");
171 const void* av[] = {
"-input",file_name,0};
172 return m_detDesc.apply(
"DD4hep_RootLoader",2,(
char**)av);
174 printout(WARNING,
"DD4hepUI",
"++ importROOT: No valid output file name supplied");
180 if ( 0 == gApplication ) {
181 std::pair<int, char**> a(argc,argv);
182 gApplication =
new TRint(
"DD4hepUI", &a.first, a.second);
183 printout(INFO,
"DD4hepUI",
"++ Created ROOT interpreter instance for DD4hepUI.");
186 printout(WARNING,
"DD4hepUI",
187 "++ Another ROOT application instance already exists. Keep existing instance.");
193 if ( 0 != gApplication ) {
194 if ( !gApplication->IsRunning() ) {
198 except(
"DD4hepUI",
"++ The ROOT application is already running.");
200 except(
"DD4hepUI",
"++ No ROOT interpreter instance present!");
Handle< NamedObject > alignmentMgr() const
Install the dd4hep alignment manager object.
long importROOT(const char *file_name) const
Import the entire detector description object from a root file.
virtual void fromXML(const std::string &fname, DetectorBuildType type=BUILD_DEFAULT) const
Detector interface: Read any geometry description or alignment file.
virtual void redrawSubtree(const char *path) const
Detector interface: Re-draw the entire sub-tree scene.
virtual long apply(const char *factory, int argc, char **argv) const
Detector interface: Manipulate geometry using factory converter.
bool isValid() const
Check the validity of the object held by the handle.
virtual long dumpDet(const char *path=0) const
Dump the DetElement tree with placement volumes.
Detector * instance() const
Access to the Detector instance.
PrintLevel setPrintLevel(PrintLevel level) const
Set the printout level from the interactive prompt.
int setVisLevel(int level)
Set the visualization level when invoking the display.
long runInterpreter() const
Execute ROOT interpreter instance.
virtual long dumpVols(int argc=0, char **argv=0) const
Dump the volume tree.
virtual void drawSubtree(const char *path) const
Detector interface: Draw detector sub-tree the scene on a OpenGL pane.
DD4hepUI(Detector &instance)
Default constructor.
virtual void redraw() const
Detector interface: Re-draw the entire scene.
Detector * detectorDescription() const
Access to the Detector instance.
virtual long dumpDetMaterials(const char *path=0) const
Dump the DetElement tree with volume materials.
virtual void draw() const
Detector interface: Draw the scene on a OpenGL pane.
DetectorBuildType
Detector description build types.
Namespace for the AIDA detector description toolkit.
virtual ~DD4hepUI()
Default destructor.
The main interface to the dd4hep detector description package.
long loadConditions(const std::string &fname) const
Load conditions from file.
long saveROOT(const char *file_name) const
Dump the entire detector description object to a root file.
Handle< NamedObject > conditionsMgr() const
Install the dd4hep conditions manager object.
long createInterpreter(int argc, char **argv)
Create ROOT interpreter instance.
virtual long dumpStructure(const char *path=0) const
Dump the raw DetElement tree.