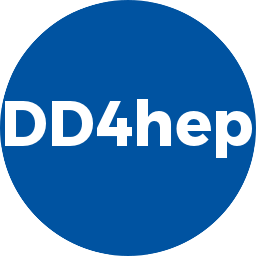 |
DD4hep
1.31.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
13 #ifndef DD4HEP_MATRIXHELPERS_H
14 #define DD4HEP_MATRIXHELPERS_H
20 class TGeoTranslation;
37 ROOT::Math::XYZVector
_scale(
const TGeoMatrix* matrix);
39 ROOT::Math::XYZVector
_scale(
const TGeoMatrix& matrix);
132 int _matrixEqual(
const TGeoMatrix& left,
const TGeoMatrix& right);
137 #endif // DD4HEP_MATRIXHELPERS_H
TGeoTranslation * _translation(const Position &pos)
Convert a Position object to a TGeoTranslation.
void _decompose(const TGeoMatrix &matrix, Position &pos, Rotation3D &rot)
Decompose a generic ROOT Matrix into a translation (Position) and a Rotation3D.
TGeoRotation * _rotationZYX(const RotationZYX &rot)
Convert a RotationZYX object to a newly created TGeoRotation.
ROOT::Math::XYZVector XYZAngles
ROOT::Math::XYZVector _scale(const TGeoMatrix *matrix)
Extract the scale part of a TGeoMatrix.
TGeoIdentity * _identity()
Access the TGeo identity transformation.
TGeoHMatrix * _transform(const Transform3D &trans)
Convert a Transform3D object to a newly created TGeoHMatrix.
TGeoRotation * _rotation3D(const Rotation3D &rot)
Convert a Rotation3D object to a newly createdTGeoRotation.
ROOT::Math::Rotation3D Rotation3D
ROOT::Math::Translation3D Translation3D
@ MATRICES_DIFFER_ROTATION
XYZAngles _xyzAngles(const double *matrix)
Convert a 3x3 rotation matrix to XYZAngles.
ROOT::Math::Transform3D Transform3D
ROOT::Math::XYZVector Position
@ MATRICES_DIFFER_TRANSLATION
Namespace for the AIDA detector description toolkit.
int _matrixEqual(const TGeoMatrix &left, const TGeoMatrix &right)
Check matrices for equality.
ROOT::Math::RotationZYX RotationZYX
double determinant(const TGeoMatrix *matrix)
Access determinant of rotation component (0 if no rotation present)