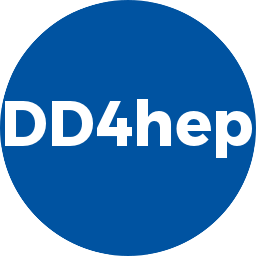 |
DD4hep
1.32.1
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
13 #ifndef DDG4_IOSTREAMS_H
14 #define DDG4_IOSTREAMS_H
20 #pragma GCC diagnostic push
21 #pragma GCC diagnostic ignored "-Wshadow" // Code that causes warning goes here
25 #include <boost/iostreams/categories.hpp>
26 #include <boost/iostreams/detail/ios.hpp>
27 #include <boost/iostreams/detail/path.hpp>
28 #include <boost/iostreams/positioning.hpp>
32 #define __DD4HEP_LOCAL_GNUC__ __GNUC__
34 #include <boost/iostreams/stream.hpp>
35 #define __GNUC__ __DD4HEP_LOCAL_GNUC__
37 #include <boost/iostreams/stream.hpp>
82 struct category : boost::iostreams::seekable_device_tag, boost::iostreams::closable_tag { };
95 void open(
const char* path, BOOST_IOS::openmode mode = BOOST_IOS::in | BOOST_IOS::out );
139 struct category : boost::iostreams::input_seekable,
140 boost::iostreams::device_tag,
141 boost::iostreams::closable_tag { };
170 void open(
const char* path, BOOST_IOS::openmode mode = BOOST_IOS::in)
174 void open(
const std::string& path, BOOST_IOS::openmode mode = BOOST_IOS::in)
175 {
open(path.c_str(), mode); }
178 template<
typename Path>
void open(
const Path& path, BOOST_IOS::openmode mode = BOOST_IOS::in)
179 {
open(detail_path(path), mode); }
200 template <
typename T>
201 class dd4hep_file_sink :
private dd4hep_file<T> {
205 struct category : boost::iostreams::output_seekable,
206 boost::iostreams::device_tag,
207 boost::iostreams::closable_tag { };
228 explicit dd4hep_file_sink(
const std::string& path, BOOST_IOS::openmode mode = BOOST_IOS::out)
236 template<
typename Path>
245 void open(
const std::string& path, BOOST_IOS::openmode mode = BOOST_IOS::out )
246 {
open(path.c_str(),mode); }
249 void open(
const char* path, BOOST_IOS::openmode mode = BOOST_IOS::out )
253 template<
typename Path>
void open(
const Path& path, BOOST_IOS::openmode mode = BOOST_IOS::out )
254 {
open(detail_path(path), mode); }
260 #pragma GCC diagnostic pop
263 #endif // DDG4_IOSTREAMS_H
boost::iostreams::detail::path detail_path
void open(handle_type fd, dd4hep_file_flags flags)
open overloads taking file descriptors
void open(const char *path, BOOST_IOS::openmode mode=BOOST_IOS::out)
open overload taking C-style string
dd4hep_file_sink()
Default constructor.
dd4hep file sink extension to boost::iostreams
dd4hep_file(handle_type fd, dd4hep_file_flags)
Constructors taking file desciptors.
bool is_open() const
Check if the file stream is opened.
dd4hep_file_sink(const std::string &path, BOOST_IOS::openmode mode=BOOST_IOS::out)
Constructor taking a std::string.
std::streamsize read(char_type *s, std::streamsize n)
Read from input stream.
dd4hep_file_sink(const dd4hep_file_sink< T > &other)
Copy constructor.
dd4hep_file_sink(handle_type fd, dd4hep_file_flags flags)
Constructors taking file desciptors.
void open(const std::string &path, BOOST_IOS::openmode mode=BOOST_IOS::in)
open overload taking a std::string
descriptor::char_type char_type
dd4hep_file< T > descriptor
void open(handle_type fd, dd4hep_file_flags flags)
Default destructor.
Helper structure to define boost::iostreams.
descriptor::handle_type handle_type
std::streampos seek(stream_offset off, BOOST_IOS::seekdir way)
Direct access: set file pointer of the stream.
std::streamsize write(const char_type *s, std::streamsize n)
Write to output stream.
void open(const char *path, BOOST_IOS::openmode mode=BOOST_IOS::in|BOOST_IOS::out)
open overload taking C-style string
boost::iostreams::stream_offset stream_offset
void close()
Close the file stream.
void open(const Path &path, BOOST_IOS::openmode mode=BOOST_IOS::in)
open overload taking a Boost.Filesystem path
dd4hep_file_source(const char *name, BOOST_IOS::openmode mode=BOOST_IOS::in)
Constructors taking file desciptors.
void open(const Path &path, BOOST_IOS::openmode mode=BOOST_IOS::out)
open overload taking a Boost.Filesystem path
dd4hep_file_sink(const char *path, BOOST_IOS::openmode mode=BOOST_IOS::out)
Constructor taking a C-style string.
dd4hep_file_source(const dd4hep_file_source< T > &other)
Copy constructor.
dd4hep_file()=default
Default constructor.
dd4hep_file_source(handle_type h, dd4hep_file_flags flags)
Constructors taking file desciptors.
dd4hep_file< T > descriptor
descriptor::handle_type handle_type
dd4hep_file_flags m_flag
Stream flag(s)
handle_type handle() const
Access to native stream handle.
dd4hep file source extension to boost::iostreams
handle_type m_handle
Native stream handle.
void open(const char *path, BOOST_IOS::openmode mode=BOOST_IOS::in)
open overload taking C-style string
void open(const std::string &path, BOOST_IOS::openmode mode=BOOST_IOS::out)
open overload taking a std::string
Namespace for the AIDA detector description toolkit.
dd4hep_file_source()
Default Constructor.
Helper structure to define boost::iostreams.
descriptor::char_type char_type
Helper structure to define boost::iostreams.
dd4hep file handling extension to boost::iostreams
dd4hep_file_sink(const Path &path, BOOST_IOS::openmode mode=BOOST_IOS::out)
Constructor taking a Boost.Filesystem path.
dd4hep_file(const char *fname, BOOST_IOS::openmode mode)
Constructors taking file desciptors.
void open(handle_type h, dd4hep_file_flags flags)
open overload taking file desciptors