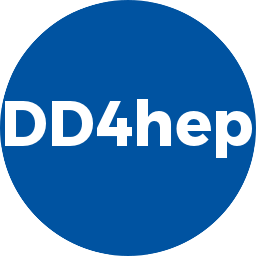 |
DD4hep
1.32.1
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
12 #ifndef DDSEGMENTATION_BITFIELD64_H
13 #define DDSEGMENTATION_BITFIELD64_H 1
25 namespace DDSegmentation {
154 void setValue(
unsigned low_Word,
unsigned high_Word ) {
155 setValue( ( low_Word & 0xffffffffULL ) | ( ( high_Word & 0xffffffffULL ) << 32 ) ) ;
241 using DDSegmentation::BitField64 ;
BitFieldValue operator[](size_t idx)
FieldID value(CellID id) const
Calculate Field value given an external 64 bit bitmap value.
Helper class for BitFieldCoder that corresponds to one field value.
unsigned highestBit() const
Lightweight helper class for BitField64 that corresponds to one field value.
Helper class for decoding and encoding a bit field of 64bits for convenient declaration.
BitField64 & operator()(CellID val)
friend std::ostream & operator<<(std::ostream &os, const BitField64 &b)
size_t index(const std::string &name) const
A bit field of 64bits that allows convenient declaration.
std::string fieldDescription() const
FieldID value(CellID bitfield) const
calculate this field's value given an external 64 bit bitmap
void setValue(unsigned low_Word, unsigned high_Word)
BitFieldValue operator[](const std::string &name)
unsigned highestBit() const
std::string valueString() const
void setValue(CellID value)
void set(CellID &bitfield, FieldID value) const
const std::string & name() const
BitFieldValue(CellID &bitfield, const BitFieldElement &bv)
only c'tor with reference to bitfield and BitFieldElement
BitFieldValue & operator=(FieldID in)
BitField64(const std::string &initString)
const BitFieldElement & _bv
size_t index(const std::string &name) const
const BitFieldCoder * _coder
unsigned highWord() const
Namespace for the AIDA detector description toolkit.
std::string fieldDescription() const
const std::string & name() const
std::ostream & operator<<(std::ostream &os, const BitField64 &b)
BitField64(const BitFieldCoder *coder)
Initialize from existing BitFieldCoder.