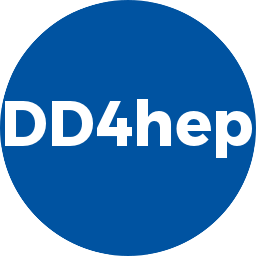 |
DD4hep
1.32.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
13 #ifndef DDEVE_DDG4EVENTHANDLER_H
14 #define DDEVE_DDG4EVENTHANDLER_H
40 typedef std::map<std::string,std::pair<TBranch*,void*> >
Branches;
42 typedef void* (*HitAccessor_t)(
void*,
DDEveHit*);
75 virtual bool Open(
const std::string& type,
const std::string& file_name)
override;
81 virtual bool GotoEvent(
long event_number)
override;
89 #endif // DDEVE_DDG4EVENTHANDLER_H
Event data actor base class for particles. Used to extract data from concrete classes.
DDEve event classes: Basic hit.
virtual bool Open(const std::string &type, const std::string &file_name) override
Open new data file.
std::pair< TFile *, TTree * > m_file
Reference to data file.
Event handler base class: Interface to all DDEve I/O actions.
Event I/O handler class for the dd4hep event display.
Int_t ReadEvent(Long64_t n)
Load the specified event.
virtual bool GotoEvent(long event_number) override
Goto a specified event in the file.
Event data actor base class for hits. Used to extract data from concrete classes.
virtual bool PreviousEvent() override
User overloadable function: Load the previous event.
virtual size_t collectionLoop(const std::string &collection, DDEveHitActor &actor) override
Call functor on hit collection.
ParticleAccessor_t m_particleConverter
Function pointer to interprete particles.
virtual const TypedEventCollections & data() const override
Access the map of simulation data collections.
std::map< std::string, std::pair< TBranch *, void * > > Branches
HitAccessor_t m_simhitConverter
Function pointer to interprete hits.
Branches m_branches
Branch map.
virtual ~DDG4EventHandler()
Default destructor.
virtual long numEvents() const override
Access the number of events on the current input data source (-1 if no data source connected)
std::string datasourceName() const override
Access the data source name.
void *(* ParticleAccessor_t)(void *, DDEveParticle *)
TypedEventCollections m_data
Data collection map.
void *(* HitAccessor_t)(void *, DDEveHit *)
ClassDefOverride(DDG4EventHandler, 0)
Namespace for the AIDA detector description toolkit.
virtual bool NextEvent() override
User overloadable function: Load the next event.
virtual CollectionType collectionType(const std::string &collection) const override
Access to the collection type by name.
Data structure to store the MC particle information.
std::map< std::string, std::vector< Collection > > TypedEventCollections
Types collection: collections are grouped by type (class name)
DDG4EventHandler()
Standard constructor.
Long64_t m_entry
File entry number.