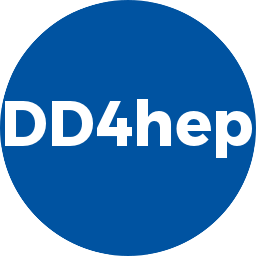 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
13 #ifndef DD4HEP_CONDITIONSDATA_H
14 #define DD4HEP_CONDITIONSDATA_H
56 typedef std::map<std::string, OpaqueDataBlock>
Params;
69 template <
typename T> T*
option()
const {
78 Params::const_iterator i=std::begin(
params);
79 if ( i != std::end(
params) )
return (*i);
80 throw std::runtime_error(
"AbstractMap: Failed to access non-existing first parameter");
84 Params::iterator i=std::begin(
params);
85 if ( i != std::end(
params) )
return (*i);
86 throw std::runtime_error(
"AbstractMap: Failed to access non-existing first parameter");
89 template <
typename T>
const T&
first()
const {
90 Params::const_iterator i=std::begin(
params);
91 if ( i != std::end(
params) )
return (*i).second.get<T>();
92 throw std::runtime_error(
"AbstractMap: Failed to access non-existing first item");
95 template <
typename T> T&
first() {
96 Params::iterator i=std::begin(
params);
97 if ( i != std::end(
params) )
return (*i).second.get<T>();
98 throw std::runtime_error(
"AbstractMap: Failed to access non-existing first item");
101 template <
typename T>
const T&
operator[](
const std::string& item)
const {
102 Params::const_iterator i=
params.find(item);
103 if ( i != std::end(
params) )
return (*i).second.get<T>();
104 throw std::runtime_error(
"AbstractMap: Failed to access non-existing item:"+item);
107 template <
typename T> T&
operator[](
const std::string& item) {
108 Params::iterator i=
params.find(item);
109 if ( i != std::end(
params) )
return (*i).second.get<T>();
110 throw std::runtime_error(
"AbstractMap: Failed to access non-existing item:"+item);
113 template <
typename T>
const T&
get(
const std::string& item)
const {
114 Params::const_iterator i=
params.find(item);
115 if ( i != std::end(
params) )
return (*i).second.get<T>();
116 throw std::runtime_error(
"AbstractMap: Failed to access non-existing item:"+item);
119 template <
typename T> T&
get(
const std::string& item) {
120 Params::iterator i=
params.find(item);
121 if ( i != std::end(
params) )
return (*i).second.get<T>();
122 throw std::runtime_error(
"AbstractMap: Failed to access non-existing item:"+item);
128 #endif // DD4HEP_CONDITIONSDATA_H
AbstractMap()
Default constructor.
AlignmentCondition::Object * cond
Params::value_type & firstParam()
Simplify access to first item of the parameter list.
const T & first() const
Simplify access to first item of the parameter list (const access)
T & first()
Simplify access to first item of the parameter list.
T * option() const
Simplify access to client data.
virtual ~AbstractMap()
Default destructor.
const Params::value_type & firstParam() const
Simplify access to first item of the parameter list (const access)
const T & get(const std::string &item) const
Simplify access to mapped item of the parameter list (const access)
size_t size() const
Access the number of contained blocks.
AbstractMap & operator=(const AbstractMap &c)
Assignment operator.
T & get(const std::string &item)
Simplify access to mapped item of the parameter list.
const T & operator[](const std::string &item) const
Simplify access to mapped item of the parameter list (const access)
virtual ~ClientData()
Default destructor.
T & operator[](const std::string &item)
Simplify access to mapped item of the parameter list.
Namespace for the AIDA detector description toolkit.
std::map< std::string, OpaqueDataBlock > Params
Conditions data block. Internaly maps other objects to abstract data blocks.