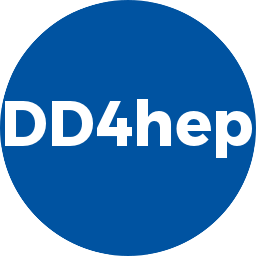 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file. 1 #ifndef GAUDIPLUGINSERVICE_GAUDI_DETAILS_PLUGINSERVICEDETAILSV1_H
2 #define GAUDIPLUGINSERVICE_GAUDI_DETAILS_PLUGINSERVICEDETAILSV1_H
28 namespace PluginService {
38 template <
typename S,
typename... Args>
39 static typename S::ReturnType
create( Args&&... args ) {
40 return new T( std::forward<Args>( args )... );
47 void*
getCreator(
const std::string&
id,
const std::string& type );
67 p2p.src =
getCreator(
id,
typeid( F ).name() );
74 std::string
demangle(
const std::type_info&
id );
91 FactoryInfo( std::string lib,
void* p =
nullptr, std::string t =
"", std::string rt =
"",
93 : library(
std::move( lib ) )
95 , type(
std::move( t ) )
96 , rtype(
std::move( rt ) )
97 , className(
std::move( cn ) )
98 , properties(
std::move( props ) ) {}
108 properties[k] = std::move(
v );
120 template <
typename F,
typename T,
typename I>
123 typename F::FuncType src;
127 std::ostringstream o;
129 return add( o.str(), p2p.dst,
typeid(
typename F::FuncType ).name(),
130 typeid(
typename F::ReturnType ).name(), demangle<T>() );
134 void* get(
const std::string&
id,
const std::string& type )
const;
137 const FactoryInfo& getInfo(
const std::string&
id )
const;
140 Registry& addProperty(
const std::string&
id,
const std::string& k,
const std::string&
v );
143 std::set<KeyType> loadedFactoryNames()
const;
147 if ( !m_initialized )
const_cast<Registry*
>( this )->initialize();
162 FactoryInfo& add(
const std::string&
id,
void* factory,
const std::string& type,
const std::string& rtype,
163 const std::string& className,
const Properties& props = Properties() );
167 if ( !m_initialized ) initialize();
193 inline void info(
const std::string& msg ) { report( Info, msg ); }
194 inline void debug(
const std::string& msg ) { report(
Debug, msg ); }
195 inline void warning(
const std::string& msg ) { report( Warning, msg ); }
196 inline void error(
const std::string& msg ) { report( Error, msg ); }
199 virtual void report( Level lvl,
const std::string& msg );
218 #define _PS_V1_INTERNAL_FACTORY_REGISTER_CNAME( name, serial ) _register_##_##serial
220 #define _PS_V1_INTERNAL_DECLARE_FACTORY_WITH_CREATOR( type, typecreator, id, factory, serial ) \
222 class _PS_V1_INTERNAL_FACTORY_REGISTER_CNAME( type, serial ) { \
224 typedef factory s_t; \
225 typedef typecreator f_t; \
226 static s_t::FuncType creator() { return &f_t::create<s_t>; } \
227 _PS_V1_INTERNAL_FACTORY_REGISTER_CNAME( type, serial )() { \
228 using ::Gaudi::PluginService::v1::Details::Registry; \
229 Registry::instance().add<s_t, type>( id, creator() ); \
231 } _PS_V1_INTERNAL_FACTORY_REGISTER_CNAME( s_##type, serial ); \
234 #define _PS_V1_INTERNAL_DECLARE_FACTORY( type, id, factory, serial ) \
235 _PS_V1_INTERNAL_DECLARE_FACTORY_WITH_CREATOR( type, ::Gaudi::PluginService::v1::Details::Factory<type>, id, factory, \
238 #endif // GAUDIPLUGINSERVICE_GAUDI_DETAILS_PLUGINSERVICEDETAILSV1_H
FactoryInfo & add(const I &id, typename F::FuncType ptr)
Add a factory to the database.
In-memory database of the loaded factories.
void info(const std::string &msg)
bool m_initialized
Flag recording if the registry has been initialized or not.
GAUDIPS_API Logger & logger()
Return the current logger instance.
Registry(const Registry &)
Private copy constructor for the singleton pattern.
void debug(const std::string &msg)
std::map< KeyType, std::string > Properties
Type used for the properties implementation.
void error(const std::string &msg)
void setLevel(Level level)
const FactoryMap & factories() const
Return the known factories (loading the list if not yet done).
GAUDIPS_API void SetDebug(int debugLevel)
Backward compatibility with Reflex.
FactoryInfo(std::string lib, void *p=nullptr, std::string t="", std::string rt="", std::string cn="", Properties props=Properties())
GAUDIPS_API void * getCreator(const std::string &id, const std::string &type)
void warning(const std::string &msg)
FactoryInfo & addProperty(const KeyType &k, std::string v)
#define GAUDI_PLUGIN_SERVICE_V1_INLINE
Logger(Level level=Warning)
FactoryMap m_factories
Internal storage for factories.
GAUDIPS_API void setLogger(Logger *logger)
std::map< KeyType, FactoryInfo > FactoryMap
Type used for the database implementation.
static S::ReturnType create(Args &&... args)
GAUDIPS_API std::string demangle(const std::type_info &id)
std::recursive_mutex m_mutex
Mutex used to control concurrent access to the internal data.
GAUDIPS_API int Debug()
Backward compatibility with Reflex.
Simple logging class, just to provide a default implementation.
FactoryMap & factories()
Return the known factories (loading the list if not yet done).