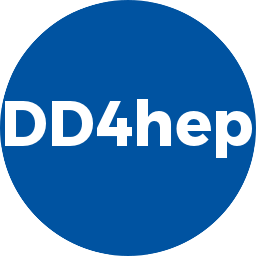 |
DD4hep
1.32.1
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
13 #ifndef DD4HEP_FILTER_H
14 #define DD4HEP_FILTER_H
43 std::vector<std::string_view>
skeys;
44 std::vector<std::regex>
keys;
45 std::unique_ptr<Filter>
next;
51 bool accepted(std::vector<std::regex>
const&, std::string_view);
55 bool isMatch(std::string_view, std::string_view);
61 std::vector<std::string_view>
split(std::string_view,
const char*);
std::vector< bool > isRegex
bool isMatch(std::string_view, std::string_view)
std::vector< std::string_view > skeys
std::unique_ptr< Filter > next
bool hasNamespace(std::string_view)
bool compareEqual(std::string_view, std::string_view)
std::vector< std::regex > keys
bool isRegex(std::string_view)
bool compareEqualCopyNumber(std::string_view, int)
bool compareEqualName(std::string_view, std::string_view)
std::vector< std::string_view > split(std::string_view, const char *)
std::vector< bool > hasNamespace
Namespace for the AIDA detector description toolkit.
std::string_view realTopName(std::string_view)
std::string_view noNamespace(std::string_view)
bool accepted(std::vector< std::regex > const &, std::string_view)